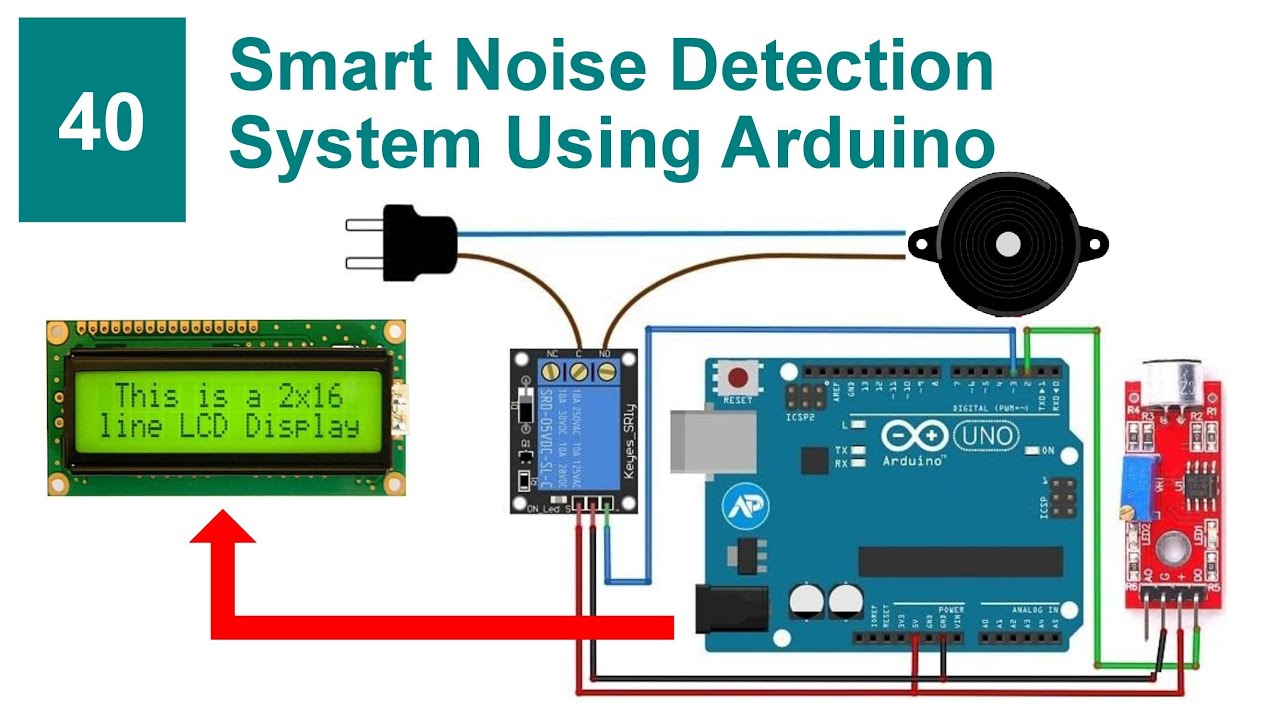
Noise Detector with Automatic Recording System
A Noise Detector with Automatic Recording System is a project that identifies loud or unusual sounds in the environment, triggers an alert, and automatically records the sound for further analysis. This type of system can be used for surveillance, wildlife monitoring, or studying noise pollution. Here’s a step-by-step guide to building such a system.
Components Needed:
- Microphone Module (e.g., MAX9814 or KY-038) – for detecting sound.
- Arduino Board (e.g., Arduino Uno or Nano) – for processing sound levels.
- MicroSD Card Module – for storing recordings.
- SD Card – to save the audio files.
- Audio Recorder Module (e.g., ISD1820) – for recording sound.
- Buzzer or LED – to alert when noise is detected.
- Jumper Wires – for connections.
- Breadboard – for assembling the components.
- Power Supply – USB cable or battery pack.
Working Principle:
- Sound Detection: The microphone module picks up ambient sounds and converts them into electrical signals. These signals are processed to measure their intensity.
- Threshold Comparison: The Arduino compares the sound level to a predefined threshold. If the noise level exceeds the threshold, the system is triggered.
- Alert and Recording: The buzzer or LED alerts users, and the audio recorder module starts recording automatically.
- Data Storage: The recorded audio is saved on the SD card for later review.
Circuit Connections:
- Microphone Module:
- Connect the VCC pin of the microphone to the 5V pin of the Arduino.
- Connect the GND pin to the ground (GND).
- Connect the OUT pin to an analog input pin (e.g., A0) on the Arduino.
- Buzzer/LED:
- Connect the positive leg of the buzzer or LED to a digital pin (e.g., D8).
- Connect the negative leg to GND (with a resistor for the LED).
- MicroSD Card Module:
- Connect the VCC to 5V, GND to ground.
- Connect the CS, MOSI, MISO, and SCK pins to their respective SPI pins on the Arduino (consult your Arduino’s pinout).
- Audio Recorder Module:
- Connect the VCC to 5V and GND to ground.
- Connect the trigger pin to a digital output pin (e.g., D9).
Code Example:
Here’s a simple Arduino code to detect noise and trigger recording:
#include <SD.h>
const int micPin = A0; // Microphone pin
const int buzzerPin = 8; // Buzzer or LED pin
const int recorderPin = 9; // Audio recorder trigger pin
const int chipSelect = 10; // SD card module CS pin
int threshold = 300; // Noise threshold value
void setup() {
pinMode(micPin, INPUT);
pinMode(buzzerPin, OUTPUT);
pinMode(recorderPin, OUTPUT);
Serial.begin(9600);
if (!SD.begin(chipSelect)) {
Serial.println("SD card initialization failed!");
while (1);
}
Serial.println("SD card initialized.");
}
void loop() {
int soundLevel = analogRead(micPin);
Serial.println(soundLevel);
if (soundLevel > threshold) {
digitalWrite(buzzerPin, HIGH); // Trigger alert
digitalWrite(recorderPin, HIGH); // Start recording
// Save a dummy file to indicate recording
File dataFile = SD.open("record.txt", FILE_WRITE);
if (dataFile) {
dataFile.println("Recording triggered due to high noise levels.");
dataFile.close();
} else {
Serial.println("Error writing to SD card.");
}
delay(5000); // Record for 5 seconds
digitalWrite(recorderPin, LOW); // Stop recording
digitalWrite(buzzerPin, LOW); // Stop alert
}
delay(100);
}
How It Works:
- The microphone module measures the sound intensity and sends data to the Arduino.
- If the sound exceeds the set threshold, the Arduino triggers:
- A buzzer or LED for an alert.
- The audio recorder module to record sound.
- An entry to be written on the SD card for event tracking.
- The system stops recording after a fixed duration.
Applications:
- Home Security: Detect break-ins or unusual sounds.
- Noise Pollution Monitoring: Record and analyze environmental noise.
- Wildlife Research: Monitor animal sounds for behavioral studies.
This system combines sound detection, real-time alerts, and automatic recording into one compact and efficient design, making it versatile for various applications.