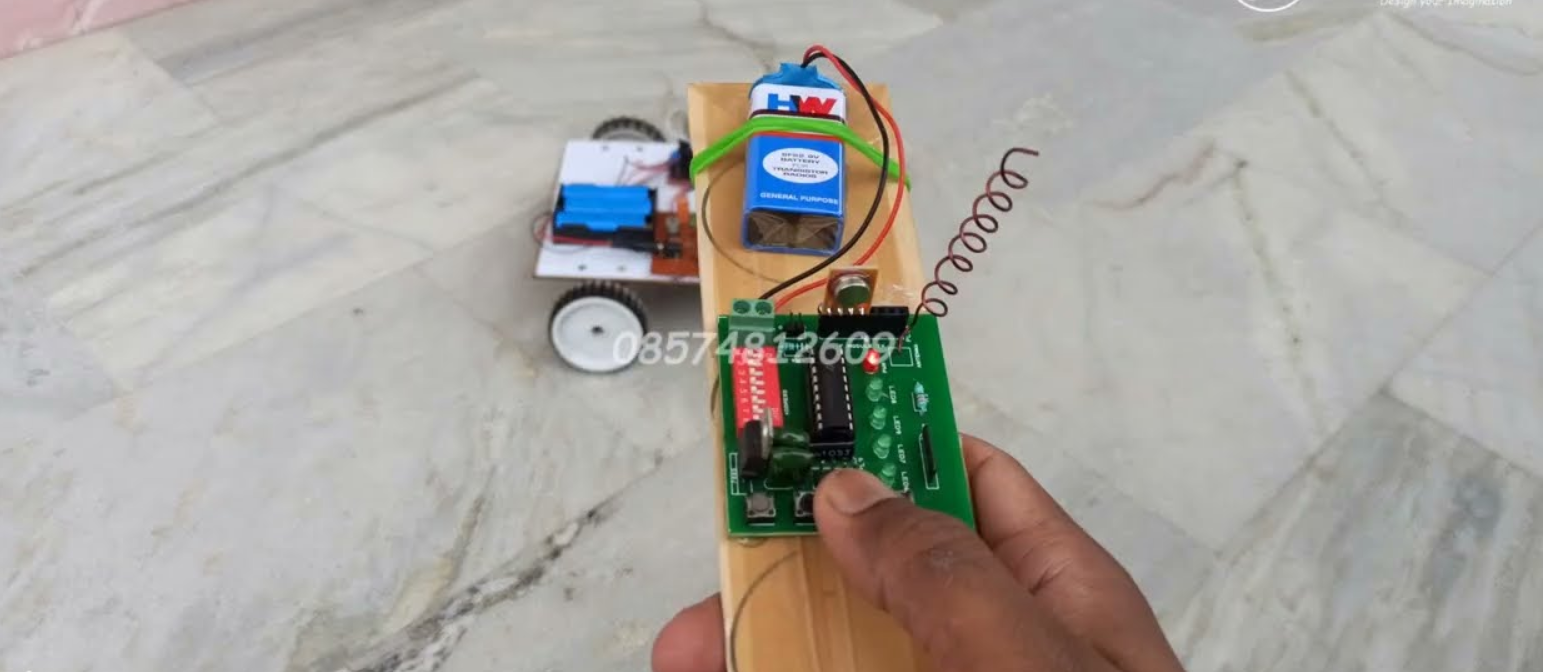
Arduino-Based RF Controlled Robot
An Arduino-Based RF Controlled Robot is a fascinating project that uses radio frequency (RF) modules to wirelessly control a robot’s movement. This type of robot is ideal for applications such as remote-controlled vehicles, surveillance, or basic robotics competitions. Here’s how to create one step-by-step.
Key Features of the Project
- Wireless Control: Operate the robot remotely using RF communication.
- Simple Design: Uses readily available RF modules for easy implementation.
- Customizable: Add sensors or features like a camera for advanced functionality.
Materials Needed
- Arduino Board (e.g., Arduino Uno or Nano)
- RF Transmitter and Receiver Module (e.g., 433 MHz modules)
- HT12E Encoder and HT12D Decoder ICs
- Joystick Module or Push Buttons (for remote control)
- DC Motors and Motor Driver Module (e.g., L298N or L293D)
- Robot Chassis (with wheels and motor mounts)
- Battery Pack (for the robot and remote)
- Jumper Wires
- Breadboard (optional for prototyping)
Step-by-Step Instructions
1. Remote Controller Setup
The remote controller sends signals to the robot via the RF transmitter.
- Connect the Joystick or Push Buttons:
- Connect the joystick or push buttons to the encoder IC (HT12E).
- The encoder converts the button presses or joystick signals into a format suitable for RF transmission.
- Connect the RF Transmitter:
- Connect the data pin of the encoder to the data input pin of the RF transmitter.
- Power the transmitter using a 5V supply and ground connection.
- Power the Remote:
- Use a 9V battery or a suitable power source to power the circuit.
2. Robot Receiver Setup
The robot receives signals from the remote via the RF receiver.
- Connect the RF Receiver:
- Connect the RF receiver to the decoder IC (HT12D).
- The decoder interprets the received signals and outputs them to control the motors.
- Motor Driver Connection:
- Connect the output of the decoder IC to the inputs of the motor driver module (e.g., L298N).
- The motor driver controls the direction and speed of the DC motors.
- Connect the Motors:
- Attach the DC motors to the motor driver’s output terminals.
- Power the Robot:
- Use a battery pack to power the Arduino and motors. Ensure sufficient voltage and current for the motors.
3. Arduino Code for the Receiver
The Arduino processes the decoded signals to control the robot’s motors. Here’s an example code:
const int motor1Pin1 = 3;
const int motor1Pin2 = 4;
const int motor2Pin1 = 5;
const int motor2Pin2 = 6;
void setup() {
pinMode(motor1Pin1, OUTPUT);
pinMode(motor1Pin2, OUTPUT);
pinMode(motor2Pin1, OUTPUT);
pinMode(motor2Pin2, OUTPUT);
}
void loop() {
int forwardSignal = digitalRead(7); // Signal for forward movement
int backwardSignal = digitalRead(8); // Signal for backward movement
int leftSignal = digitalRead(9); // Signal for turning left
int rightSignal = digitalRead(10); // Signal for turning right
if (forwardSignal == HIGH) {
moveForward();
} else if (backwardSignal == HIGH) {
moveBackward();
} else if (leftSignal == HIGH) {
turnLeft();
} else if (rightSignal == HIGH) {
turnRight();
} else {
stopMotors();
}
}
void moveForward() {
digitalWrite(motor1Pin1, HIGH);
digitalWrite(motor1Pin2, LOW);
digitalWrite(motor2Pin1, HIGH);
digitalWrite(motor2Pin2, LOW);
}
void moveBackward() {
digitalWrite(motor1Pin1, LOW);
digitalWrite(motor1Pin2, HIGH);
digitalWrite(motor2Pin1, LOW);
digitalWrite(motor2Pin2, HIGH);
}
void turnLeft() {
digitalWrite(motor1Pin1, LOW);
digitalWrite(motor1Pin2, HIGH);
digitalWrite(motor2Pin1, HIGH);
digitalWrite(motor2Pin2, LOW);
}
void turnRight() {
digitalWrite(motor1Pin1, HIGH);
digitalWrite(motor1Pin2, LOW);
digitalWrite(motor2Pin1, LOW);
digitalWrite(motor2Pin2, HIGH);
}
void stopMotors() {
digitalWrite(motor1Pin1, LOW);
digitalWrite(motor1Pin2, LOW);
digitalWrite(motor2Pin1, LOW);
digitalWrite(motor2Pin2, LOW);
}
Testing the Robot
- Power up the remote and the robot.
- Press the joystick or buttons on the remote to control the robot’s movement.
- Verify the robot moves forward, backward, left, or right based on the commands.
Optional Enhancements
- Add Sensors: Include ultrasonic sensors for obstacle avoidance.
- Camera Module: Attach a camera for remote surveillance.
- Speed Control: Use PWM signals for variable motor speeds.
Applications
- Surveillance: Wireless control of robots for security purposes.
- Learning Tool: Introduces RF communication and robotics.
- Competitions: Use in robotics events and challenges.
This Arduino-based RF controlled robot demonstrates wireless communication and robotics concepts in a practical, hands-on way, making it an exciting project for enthusiasts and students alike!