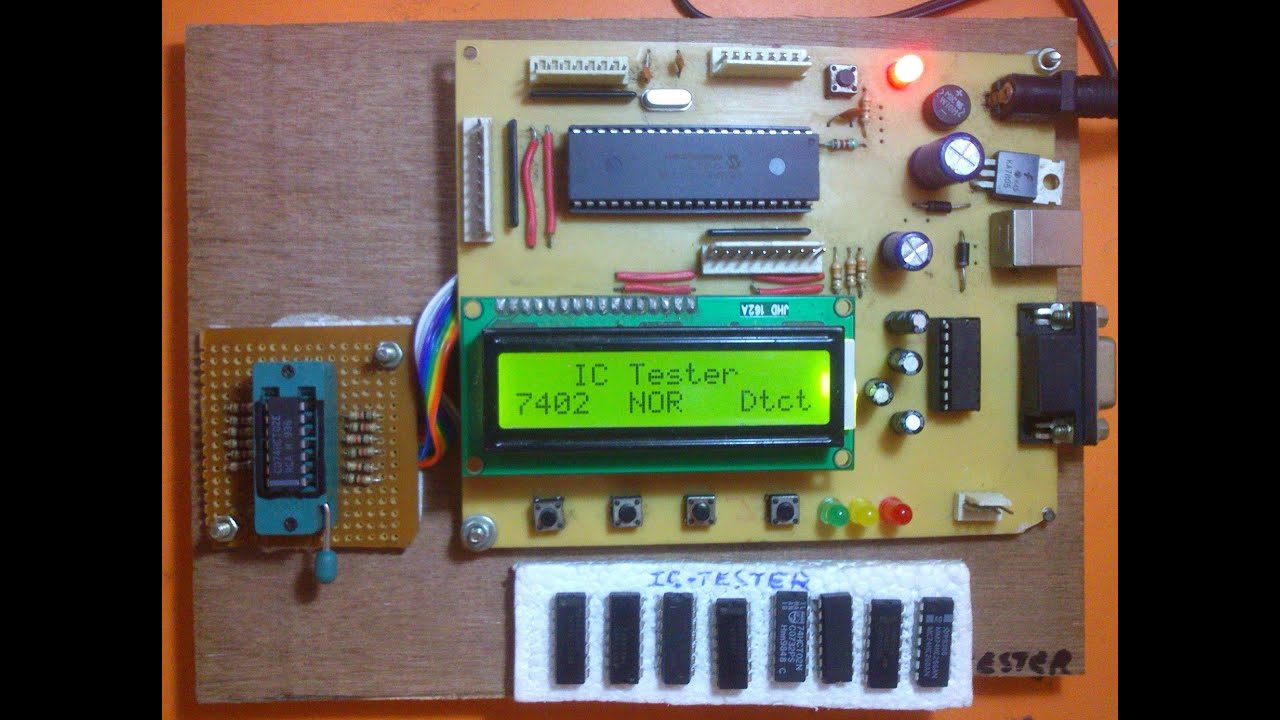
Digital IC Tester with Embedded Truth Table
How to Make a Digital IC Tester with Embedded Truth Table
A digital IC tester is a practical tool used to test the functionality of digital integrated circuits (ICs). By embedding a truth table within the tester, you can compare the IC’s outputs against expected values to verify its performance. This DIY guide will show you how to create a digital IC tester with an embedded truth table using a microcontroller.
What is a Digital IC Tester?
A digital IC tester is an electronic device that checks the functionality of digital logic ICs, such as gates, flip-flops, counters, or multiplexers. It applies test inputs to the IC and compares the outputs against a predefined truth table to determine if the IC is functioning correctly.
Why Build a Digital IC Tester?
- Cost-Effective: A DIY IC tester is more affordable than commercial ones.
- Customizable: You can program it to test specific ICs or add more functionalities.
- Portable: Small and easy to carry for fieldwork or lab use.
Components Required
- Microcontroller: Arduino Uno, Mega, or a similar board.
- 16×2 LCD Display: To display test results and IC information.
- Keypad Module: For selecting the IC type or entering commands.
- ZIF Socket: For inserting the IC under test.
- Resistors and LEDs: To create pull-up/pull-down circuits and display status.
- Power Supply: 5V DC.
- Breadboard and Wires: For prototyping.
- Push Buttons: Optional for starting the test manually.
How It Works
- The IC under test is placed in a ZIF socket.
- The microcontroller applies predefined inputs to the IC.
- The outputs are read and compared against the embedded truth table.
- The results are displayed on the LCD, indicating whether the IC is working correctly or is faulty.
Step-by-Step Guide
Step 1: Hardware Setup
- ZIF Socket Connection
- Connect the pins of the ZIF socket to the microcontroller’s digital I/O pins.
- Assign input and output pins based on the IC’s datasheet.
- LCD and Keypad Integration
- Connect the 16×2 LCD to the microcontroller using appropriate pins for data and control (e.g., RS, EN, D4-D7).
- Connect the keypad to the microcontroller for user input (e.g., selecting IC type).
- Power Supply
- Ensure the ZIF socket and connected IC receive the appropriate voltage (typically 5V for most digital ICs).
Step 2: Programming the Microcontroller
- Define the Truth Table
- Store truth tables for supported ICs in the microcontroller’s memory.
- Example: For a 7408 AND gate, the truth table is:
Input: 00, Output: 0 Input: 01, Output: 0 Input: 10, Output: 0 Input: 11, Output: 1
- Write the Test Logic
- Apply each input combination to the IC.
- Read the outputs and compare them against the stored truth table.
- Display Results
- If the outputs match the truth table, display “IC OK.”
- Otherwise, display “IC Faulty.”
Step 3: Example Code for Arduino
#includeStep 4: Testing
- Insert the IC into the ZIF socket.
- Power on the tester.
- Select the IC type using the keypad or predefined settings in the code.
- Observe the results on the LCD display.
Applications
- Test logic gates, flip-flops, counters, etc.
- Troubleshoot circuits in electronics labs.
- Verify IC functionality in embedded projects.
Tips for Enhanced Features
- Expandable Database: Use external memory or SD card to store truth tables for a large number of ICs.
- Support for Complex ICs: Add shift registers or multiplexers to test ICs with many pins.
- PC Interface: Connect to a computer via USB for detailed results and logging.